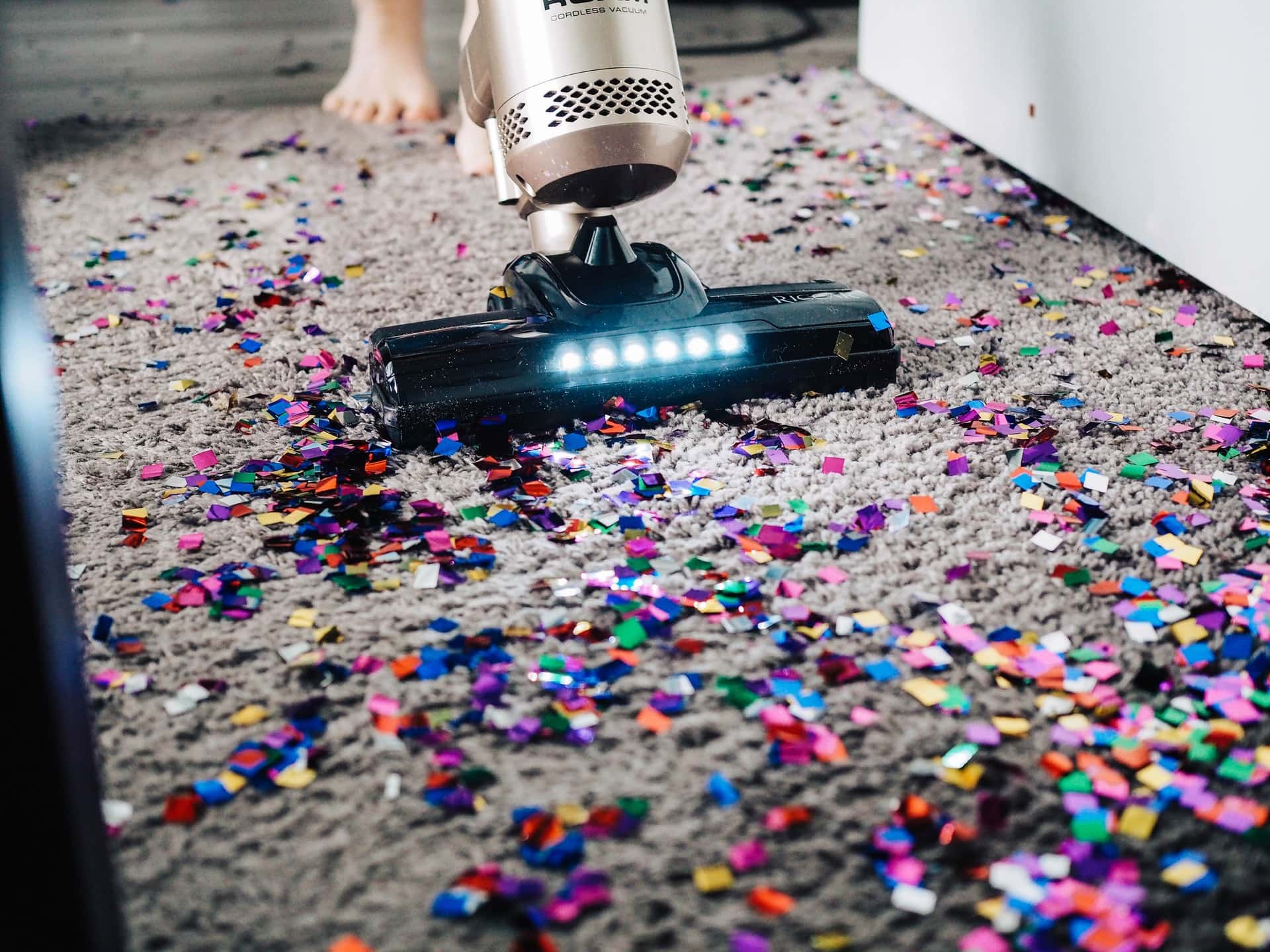
Introduction to Clean Architecture & Domain-Driven Design on PHP
PHP 7.4 was released on November 2019 with support for typed properties. Although this is just one of the improvements of the new version, that one really caught my attention. I'll tell you why I think this is so fundamentally important, but first let's understand a little about Clean Architecture and Domain-Driven Design.
Clean Architecture
Clean Architecture is a software architecture intended to keep the code under control, without all that messiness that spooks anyone from touching a code after it’s being released. It was created by Robert C. Martin, the same guy known for the Agile Manifesto and SOLID principles, which are both monumental contributions to the programming community.
Clean Architecture teaches us that what the application does, which is very unlikely to change, has to be written without any direct dependencies. That means if I change my framework, database or UI, the core of my application cannot change. External dependencies are completely replaceable. Sounds awesome, right?
Domain-Driven Design
Domain-Driven Design is hard to differentiate from Clean Architecture. DDD focus is to understand what the application should do and translate that to code, providing a lot of insights on how you should organize the business rules and data models. In theory both try to achieve the same goal but while one talks specifically about the Domain part, the other approaches the application structure directly. There are a couple differences between them and I will be talking about them later on, don’t worry about this for now.
Before we continue this discussion, I recommend that you read the Clean Architecture post on Uncle Bob’s blog and An introduction to Domain-Driven Design by Laurent Grima because they do a better job introducing these subjects than I will ever be able to.
What does it have to do with PHP?
Back to PHP, the introduction of typed properties lets you write private HttpCookie $cookie; instead of private $cookie;. Why is this important? Well, statically typing your variables makes your code safer and more readable. As Clean Architecture and DDD are all about objects and their characteristics, it allows us to finally apply their concepts in PHP language without relying on annotations.
You probably won’t understand Clean Architecture and DDD right off the bat, it takes some time to assimilate the concepts, but nothing better than learning with actual code! I wrote a simple REST API as a proof of concept combining DDD with Clean Architecture and I will be explaining each part of the code. It’s available on GitHub and works! 😃
Explaining the Proof of Concept 101: The File Structure
Let’s start by first understanding the file structure. Clean Architecture is a layered architecture, therefore in the src/ directory we have 3 directories: Domain, Infrastructure and Presentation. These are the actual layers.
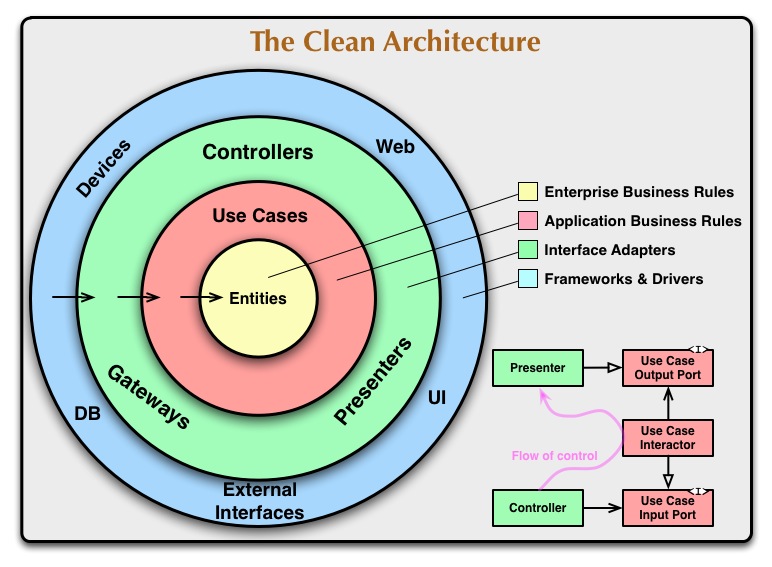
The core of the software is the Domain layer. There you’ll find the Entities, the Value Objects, the Repositories Interfaces and the Use Cases. Pretty much anything that has to do with what the application is supposed to do. It’s important to notice that in this area, everything is vanilla PHP, without the use keyword (imports) that isn’t from the Domain Layer itself.
Then we have the Infrastructure layer which is the layer where your ORM, External APIs, Validators and everything that has to do with dependencies of the application lives. They all follow what the interfaces created on the Domain layer demanded. Here’s an example:
Repository Interface (Domain Layer)
Repository Implementation (Infrastructure Layer)
Last but not least, there is the Presentation layer, responsible for the entry points of your application, so it’s where the HTTP or CLI framework will be as well as template engine if you’re also developing an user interface. Controllers, presenters and middlewares are all written in this layer. I usually separate each type of entry point in its sub-folder. That’s why on the example code everything live in the Presentation/Api directory.
If I wanted to add a CLI for example, I would create the Presentation/Cli directory and there I would install a CLI framework and write the necessary controllers, presenters and middlewares.
Conclusion
In this article you learned what Clean Architecture and DDD are all about and started to understand how to apply their concepts in PHP with the proof of concept code. In the next article we’ll be talking about the Domain Layer in more detail, stay tuned!
The Domain Layer - Clean Architecture & Domain-Driven Design on PHP »
Comments